ChatGPTは、人間のテキストを学習し、それに基づいて新しいテキストを生成する人工知能モデルです。対話型でチャット形式でやり取りが出来て、さらにはプログラミングも出来るということで、以下、ChatGPTとの対話形式で進めて行きたいと思います。
では今回は、Pythonで動くグラフを作成してもらうことにしましょう。
今回の目標
とあるEAのバックテスト結果である以下の損益グラフを材料にします。
こちらは、初期資金が100万円として、2020年1月〜2022年12月までの3年間の損益結果(残高推移)をグラフで表したものです。
これを、MT4やMT5のバックテストのグラフのように、時間経過で徐々に折線が形成されていくようなGIFデータを作成したいです。
より具体的にお願いしてみる
A:次のように matplotlib
の FuncAnimation
を使用することで、時間経過に応じた資産残高の推移を表す動的なグラフを作成することができます。
import matplotlib.pyplot as plt
import matplotlib.animation as animation
import pandas as pd
df = pd.read_csv('asset_data.csv') # replace with your data
# set index to datetime
df.index = pd.to_datetime(df['timestamp'])
df = df.drop(['timestamp'], axis=1)
fig, ax = plt.subplots()
def animate(i):
ax.clear()
ax.plot(df.index[:i+1], df['balance'][:i+1])
plt.xticks(rotation=45)
plt.xlabel('Time')
plt.ylabel('Balance')
plt.title('Asset Balance Over Time')
ani = animation.FuncAnimation(fig, animate, frames=len(df), interval=200)
plt.show()
この例では、最初にインデックスとして設定するために df.index
に pd.to_datetime
関数を使用してdatetime形式の時間を設定しています。次に、折れ線グラフを描画するために ax.plot
関数を使用しています。 animate
関数を呼び出すことによって、グラフを更新し、時間の経過に応じてグラフを更新しています。
この例を参考にして、自分のデータと要件に合わせて調整することができます。
asset_data.csvを用意する
ひとまずChatGPTが土台のコードを作成くれたので、しかもasset_data.csvを用意してというところまで形作ってくれたので、指示通りデータを用意します。
今回は、上記グラフの数値データである以下を用意しました。
これを同じフォルダ置いて実行したら、問題なく動作しました。
少しグラフが小さかったので、、、
A:次のように、グラフのサイズを調整することができます。また、yticks
関数を使用して、y軸の数値表記を100万単位に変更することができます。
import matplotlib.pyplot as plt
import matplotlib.animation as animation
import pandas as pd
df = pd.read_csv('asset_data.csv') # replace with your data
# set index to datetime
df.index = pd.to_datetime(df['timestamp'])
df = df.drop(['timestamp'], axis=1)
fig, ax = plt.subplots(figsize=(12, 8))
def animate(i):
ax.clear()
ax.plot(df.index[:i+1], df['balance'][:i+1])
plt.xticks(rotation=45)
plt.xlabel('Time')
plt.ylabel('Balance (100,000)')
plt.yticks(df['balance'][:i+1][::100000])
plt.title('Asset Balance Over Time')
ani = animation.FuncAnimation(fig, animate, frames=len(df), interval=200)
plt.show()
この例では、figsize
引数を使用して、グラフのサイズを12×8に変更しています。また、yticks
関数を使用して、y軸のメモリを100万単位に変更するように指示しています。
少しGIFの動きが遅かったので、、、
A:はい、interval
引数を使用することで、GIF の動きを速くすることができます。次のように、interval
引数を変更することで、GIF の動きを速くすることができます。
import matplotlib.pyplot as plt
import matplotlib.animation as animation
import pandas as pd
df = pd.read_csv('asset_data.csv') # replace with your data
# set index to datetime
df.index = pd.to_datetime(df['timestamp'])
df = df.drop(['timestamp'], axis=1)
fig, ax = plt.subplots(figsize=(12, 8))
def animate(i):
ax.clear()
ax.plot(df.index[:i+1], df['balance'][:i+1])
plt.xticks(rotation=45)
plt.xlabel('Time')
plt.ylabel('Balance (100,000)')
plt.yticks(df['balance'][:i+1][::100000])
plt.title('Asset Balance Over Time')
ani = animation.FuncAnimation(fig, animate, frames=len(df), interval=100)
plt.show()
この例では、interval
引数を100に変更しています。この値は、GIF の動きを表すフレーム間の間隔を指定するミリ秒単位の数値です。小さい数値を指定すると、GIF の動きが速くなります。
GIFで保存したいので、、、
はい、以下のように修正することで、y軸を10万単位の目盛りにすることができます。また、animation.FuncAnimation
の save
メソッドを使用することで、GIF として保存することができます。
import matplotlib.pyplot as plt
import matplotlib.animation as animation
import pandas as pd
df = pd.read_csv('asset_data.csv') # replace with your data
# set index to datetime
df.index = pd.to_datetime(df['timestamp'])
df = df.drop(['timestamp'], axis=1)
fig, ax = plt.subplots(figsize=(12, 8))
def animate(i):
ax.clear()
ax.plot(df.index[:i+1], df['balance'][:i+1]/100000)
plt.xticks(rotation=45)
plt.xlabel('Time')
plt.ylabel('Balance (100,000)')
plt.yticks(df['balance'][:i+1][::100000]/100000)
plt.title('Asset Balance Over Time')
ani = animation.FuncAnimation(fig, animate, frames=len(df), interval=100)
ani.save('asset_balance.gif', writer='imagemagick')
この例では、save
メソッドを使用して、GIF 形式のファイルとして asset_balance.gif
という名前で保存することができます。
もう少し目盛りを細かく、、、
A:はい、以下のように修正することで、y軸の目盛り線をもっと細かくすることができます。
import matplotlib.pyplot as plt
import matplotlib.animation as animation
import pandas as pd
df = pd.read_csv('asset_data.csv') # replace with your data
# set index to datetime
df.index = pd.to_datetime(df['timestamp'])
df = df.drop(['timestamp'], axis=1)
fig, ax = plt.subplots(figsize=(12, 8))
def animate(i):
ax.clear()
ax.plot(df.index[:i+1], df['balance'][:i+1]/100000)
plt.xticks(rotation=45)
plt.xlabel('Time')
plt.ylabel('Balance (100,000)')
plt.yticks(range(int(df['balance'][:i+1].min()/100000), int(df['balance'][:i+1].max()/100000)+1, 5000))
plt.title('Asset Balance Over Time')
ani = animation.FuncAnimation(fig, animate, frames=len(df), interval=100)
ani.save('asset_balance.gif', writer='imagemagick')
この例では、yticks
により y 軸の目盛り線を設定しています。range(int(df['balance'][:i+1].min()/100000), int(df['balance'][:i+1].max()/100000)+1, 5000)
の部分で、最小値から最大値まで5000単位で目盛り線を設定しています。
動くグラフ(GIFデータ)の完成
今回完成したグラフは以下の通りです。いかがでしょうか。目標としていたものには十分到達しています。
まとめ
以上、見てきたように、
さらには英語が出来なくても、
全て日本語でやりたいことを伝えて、
細かい調整も日本語で細かいことを伝えて修正してもらって、
目標としたものを作成する。
というChatGPTでプログラミングをする具体例を示してみたくて、以上のようなやり取りでご紹介させていただきました。
最後に、以下のような質問をしてみます。
A:このコードは、pandasのDataFrame形式のインプットデータを用いて、時間経過に応じた資産残高の推移を表す折れ線グラフを作成し、動的にアニメーション化するものです。
- 最初に、インプットデータ(CSVファイル)を読み込んで、DataFrame形式に変換します。
- 次に、DataFrameのインデックスをdatetime形式の時間に設定します。
- 折れ線グラフを作成するための、matplotlibのFigureとAxesオブジェクトを作成します。
- 次に、アニメーション用にグラフを更新するための animate 関数を定義します。この関数は、毎回グラフを消去して、最新のデータで再描画する役割を持ちます。
- 最後に、animate関数を使ってグラフをアニメーション化し、gif形式で保存します。
このコードにより、時間経過に応じた資産残高の推移を表す動的なグラフが作成されます。
ChatGPTで他にも試してみた
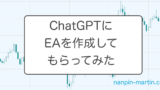
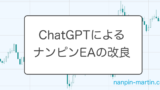
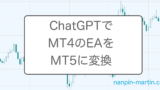
BingAIでMT5用EAを作成してみた
BingAIというチャット型AIもご紹介しています。
キャッシュバック口座の開設
作成したEAを利用して実際に運用する際は、通常の口座開設ではなく、キャッシュバック口座を開設するのがおすすめです。以下の記事で、キャッシュバックサイト経由で海外FX口座を開設する際のメリットとデメリットをまとめていますのでご覧ください。
利用者の特性に応じて、リアル口座をどのように開設するのが最適かを考える参考になると思います。